Operating System Lecture Notes - Introduction
In this note, you could find the basic definition of Operating Systems and the general architecture of computer.
Defining Operating Systems
What can an OS do for me?
File systems: where is the file physically written on the disk and how is it retrieved?
Abstraction: why looks the instruction the same independent of the device?
Concurrency: what if multiple programs access the same file simultaneously? What if an other process starts running?
Security: why is the access denied? Where in memory will the array be stored and how is it protected from unauthorised access?
What if the array requires more memory than physically available?
What if only part of the array is currently in use ?
What is part of the operating system?
Memory management, CPU scheduling, file system, communication, memory management, interrupt handling, GUI, . . .
A resource manager
- Many modern operating systems use multi-programming
to improve user experience and maximize
resource utilization
- Disks are slow: without multi-programming, CPU time is wasted while
waiting for I/O requests
- Imagine a CPU running at 3.2 GHz (approx. 3:2 \(\times\) 109 instructions per second)
- Imagine a disk rotating at 7200 RPM, taking 4.2 ms to rotate half a track
- I/O is slow, we are missing out on 3.2 \(\times\) 4.2 \(\times\) \(10^6\) instructions (13.44m)!
- Disks are slow: without multi-programming, CPU time is wasted while
waiting for I/O requests
- The implementation of multi-programming has important consequences for operating system design
- The operating system must allocate/share resources
(including CPU, memory, I/O devices) fairly and safely between competing
processes:
- In time, e.g. CPUs and printers
- In space, e.g., memory and disks
- The execution of multiple programs (processes) needs to be
interleaved with one another:
- This requires context switches and process scheduling ) \(\Rightarrow\) mutual exclusion, deadlock avoidance, protection, . .
Origin
In the early days, programmers had to deal directly with the hardware
- Real computer hardware is ugly
- Hardware is extremely difficult to manipulate/program
An operating system is a layer of indirection on top of the hardware:
- It provide abstractions for application programs (e.g., file systems)
- It provides a cleaner and easier interface to the hardware and hides the complexity of “bare metal”
- It allows the programmer to be lazy by using common routines :-)
Why study operating system?
- The programs that we write use operating system functionality
- How are the operating system’s services/abstractions implemented
Computer Architecture
CPU design
- CPU’s basic cycle consist of fetch, decode, and execute (pipelines, or superscalar)
- Every CPU has his own instruction set
- A CPU has a set of registers (extremely fast memory close to the CPU
“core”)
- Registers are used to store data and for special functions (e.g. program counter, program status word – mode bit)
- The compiler/programmer decides what to keep in the registers
- Context switching must save and restore the CPU’s internal state, including its registers
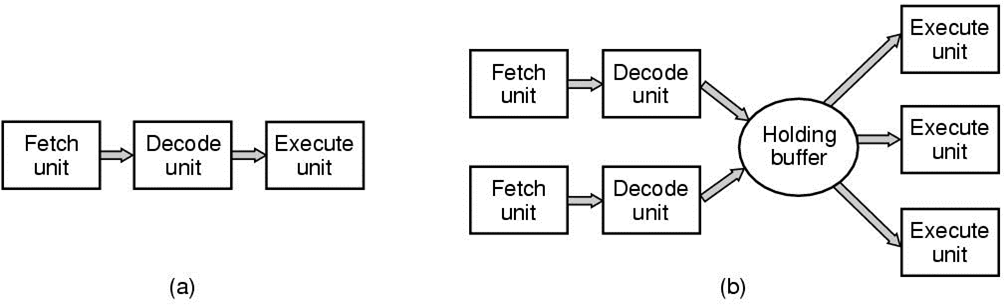
Memory management Unit
There are two different address spaces:
- the logical address space seen by the process and used by the compiler
- the physical address space seen by the hardware/OS
When compiling code, memory addresses must be assigned to variables and instructions, the compiler does not know what memory addresses will be available in physical memory
It will just assume that the code will start running at address 0 when generating the machine code
On some rare occasions, the process may run at physical address 0
- physical address = logical address + 0
On other occasions, it will be running at a completely different location in physical memory and an offset is added
- physical address = logical address + offset
The
memory management unit
(MMU) is responsible for address translation (“adding the offset”)- Different processes require different address translation (offsets)
- Context switching requires the MMU to be updated (and registers, cache, ...)
Example
1 |
|
- The same addresses will be displayed for
iVar
. The address printed on the screen is the logical address - The value for
iVar
in the first run doesn’t influence the second run’s value
Moore’s Law
“The number of transistors on an integrated circuit (chip) doubles roughly every two years”
- Closely linked, but not necessarily related to performance
- Moore’s still continuing, but the “power wall” slows performance
improvements of single core/single processor systems
- A few cores for multiple “programs” is easy to justify
- How to use massively parallel computers/CPUs/many core machines
- Can we extract parallelism automatically, can we implement parallelism at the lowest level (similar to multiprogramming)
Lead to multi-core / parallel development
Multi-core, hyperthreaded processors
Modern CPUs contain multiple cores and are often hyper-threaded
Evolution in hardware has implications on operating system design
XP did not support multi processor architectures
Process scheduling needs to account for load balancing and CPU affinity
Cache coherency becomes important (manage run-time data)
Previous exam: Describe how, in your opinion, recent developments in computer architecture and computer design have influenced operating system design
Timer Interrupts
- Interrupts temporarily pause a process’s normal operation
- Different types of interrupts exist, including:
- Timer interrupts by CPU clock
- I/O interrupts for I/O completion or error codes
- Software generated, e.g. errors and exceptions
- Context switches (i.e. switching between processes) can be initiated by timer interrupts after a “set time”
- Timer generates an interrupt
- CPU finishes current instruction and tests for interrupt
- Transfer to interrupt service routine
- Hardware saves current process state (PSW, program counter)
- Set program counter to interrupt service routine
- Save registers and other state information
- Carry out interrupt service routine (scheduler)
- Restore next process to run